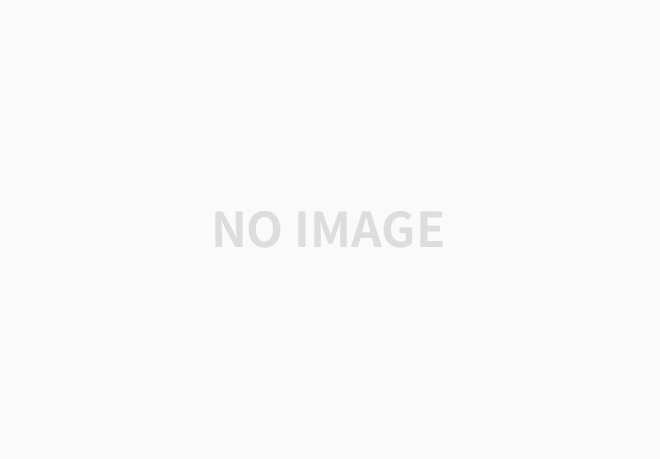
import React from 'react';
import {Modal, Pressable, StyleSheet, Text, View} from 'react-native';
import {colors, margin} from '~/constants';
const width = 200;
interface IProps {
isVisible: boolean;
text: string;
secondText?: string;
onConfirm: () => void;
onClose: () => void;
}
const Level1 = () => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
<View style={styles.horizontalConnectorLevel1} />
<View style={styles.matchContainer}>
{/* 팀1 */}
<View style={styles.teamContainerLevel1}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀1</Text>
</View>
<Level2 index={0} />
</View>
{/* 팀2 */}
<View style={styles.teamContainerLevel1}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀2</Text>
</View>
<Level2 index={1} />
</View>
</View>
</>
);
};
const Level2 = ({index}) => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
<View style={styles.horizontalConnectorLevel2} />
<View style={styles.matchContainer}>
{/* 팀1 */}
<View style={styles.teamContainerLevel2}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>팀3</Text> : <Text>팀5</Text>}
</View>
<Level3 />
</View>
{/* 팀2 */}
<View style={styles.teamContainerLevel2}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>팀4</Text> : <Text>팀6</Text>}
</View>
<OddLevel3 />
</View>
</View>
</>
);
};
const Level3 = () => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
<View style={styles.horizontalConnectorLevel3} />
<View style={styles.matchContainer}>
{/* 팀1 */}
<View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀1</Text>
</View>
</View>
{/* 팀2 */}
<View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀2</Text>
</View>
</View>
</View>
</>
);
};
const OddLevel3 = () => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.oddVerticalConnector} />
{/* 중간 연결선 */}
{/* <View style={styles.horizontalConnectorLevel3} /> */}
<View style={styles.matchContainer}>
{/* 팀1 */}
{/* <View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀1</Text>
</View>
</View> */}
{/* 팀2 */}
<View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀2</Text>
</View>
</View>
</View>
</>
);
};
const ConfirmModal = ({
isVisible,
text,
secondText,
onConfirm,
onClose,
}: IProps) => {
return (
<Modal visible={isVisible} transparent={true} onRequestClose={onClose}>
{/* <FullScreenLoader isLoading={isLoading} /> */}
<Pressable onPress={onClose} style={{flex: 1}}>
<View style={styles.modalOverlay}>
{/* 모달의 콘텐츠 부분 */}
<View style={styles.modalView}>
<View style={styles.bracket}>
{/* 최종 승자 */}
<View style={styles.team}>
<Text>우승팀</Text>
</View>
<Level1 />
</View>
</View>
</View>
</Pressable>
</Modal>
);
};
const styles = StyleSheet.create({
modalOverlay: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'rgba(255, 255, 255, 1)', // 반투명 배경
},
modalView: {
position: 'absolute',
justifyContent: 'center',
alignItems: 'center',
// width: '60%', // 모달의 폭
// width: 'auto',
width: '100%',
height: 'auto', // 모달의 높이
backgroundColor: colors.mainContainerColor,
padding: 20,
borderRadius: 10,
shadowColor: '#000',
shadowOffset: {width: 0, height: 2},
shadowOpacity: 0.25,
shadowRadius: 4,
elevation: 5,
},
verticalGreyLine: {
width: 1,
height: '100%',
borderWidth: 0.5,
borderColor: colors.greyLine,
},
bracket: {
// backgroundColor: 'red',
width: 500,
flexDirection: 'column',
alignItems: 'center',
justifyContent: 'center',
marginTop: 50,
},
matchContainer: {
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'center',
},
teamContainerLevel1: {
width: 200,
// backgroundColor: 'darkblue',
flexDirection: 'column',
// justifyContent: 'space-between',
alignItems: 'center',
},
teamContainerLevel2: {
width: 100,
// backgroundColor: 'blue',
flexDirection: 'column',
// justifyContent: 'space-between',
alignItems: 'center',
},
teamContainerLevel3: {
width: 50,
// backgroundColor: 'blue',
flexDirection: 'column',
// justifyContent: 'space-between',
alignItems: 'center',
},
team: {
backgroundColor: 'green',
padding: 10,
borderWidth: 1,
borderColor: 'black',
marginVertical: 5,
},
horizontalConnectorLevel1: {
borderTopWidth: 1,
borderColor: 'black',
width: width,
},
horizontalConnectorLevel2: {
borderTopWidth: 1,
borderColor: 'black',
width: width / 2,
},
horizontalConnectorLevel3: {
borderTopWidth: 1,
borderColor: 'black',
width: width / 4,
},
verticalConnector: {
borderLeftWidth: 1,
borderColor: 'black',
minHeight: width / 6,
},
oddVerticalConnector: {
borderLeftWidth: 1,
borderColor: 'black',
minHeight: width / 6,
},
halfConnector: {
minHeight: 25,
},
});
export default ConfirmModal;
중간에 어느팀 올라갔는지 없고 시작팀들과 우승팀만 있는것
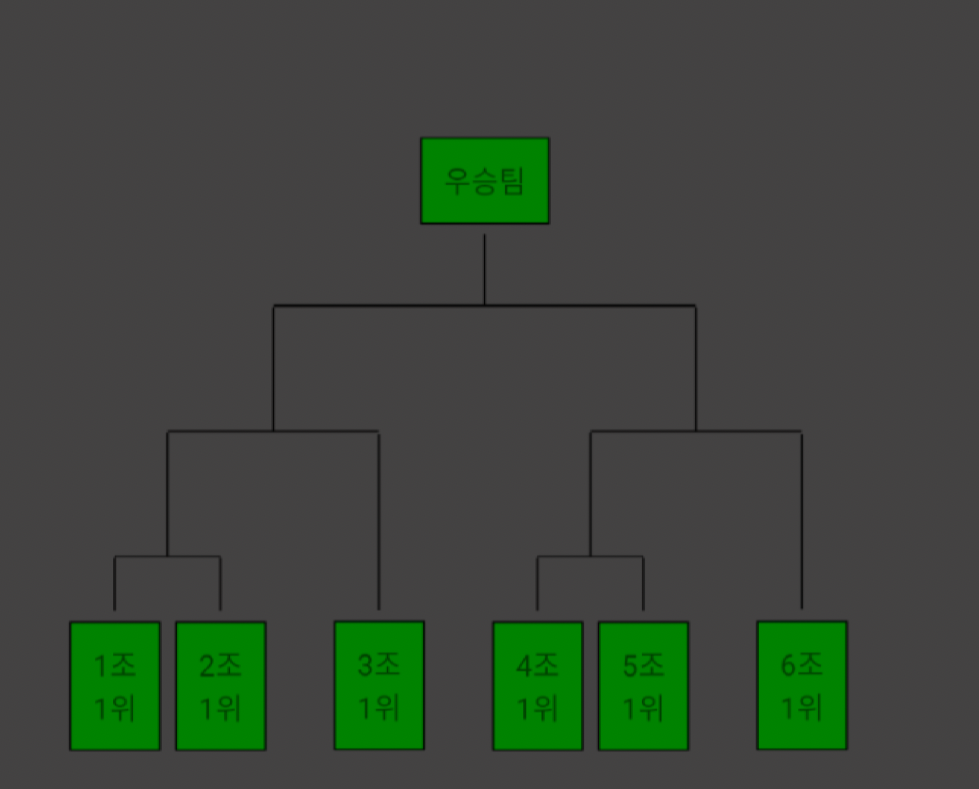
import React from 'react';
import {Modal, Pressable, StyleSheet, Text, View} from 'react-native';
import {colors, margin} from '~/constants';
const width = 200;
interface IProps {
isVisible: boolean;
text: string;
secondText?: string;
onConfirm: () => void;
onClose: () => void;
}
const options = [
{
id: 'team10_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (3팀, 3팀, 4팀)',
value: '1',
teams: [3, 3, 4],
ADVANCE_RANK: '1',
},
{
id: 'team10_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (5팀, 5팀)',
value: '2',
teams: [5, 5],
ADVANCE_RANK: '2',
},
{
id: 'team10_4',
label: '조별 리그전 이후 1,2,3위팀 진출 토너먼트 (5팀, 5팀)',
value: '24',
teams: [5, 5],
ADVANCE_RANK: '3',
},
{
id: 'team10_3',
label: '조별 리그전만 진행 (5팀, 5팀)',
value: '3',
teams: [5, 5],
ADVANCE_RANK: '0',
},
{
id: 'team12_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (3팀, 3팀, 3팀, 3팀)',
value: '4',
teams: [3, 3, 3, 3],
ADVANCE_RANK: '1',
},
{
id: 'team12_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (3팀, 3팀, 3팀, 3팀)',
value: '5',
teams: [3, 3, 3, 3],
ADVANCE_RANK: '2',
},
{
id: 'team12_3',
label: '조별 리그전 이후 1,2,3위팀 진출 토너먼트 (6팀, 6팀)',
value: '6',
teams: [6, 6],
ADVANCE_RANK: '3',
},
{
id: 'team12_4',
label: '조별 리그전만 진행 (6팀, 6팀)',
value: '7',
teams: [6, 6],
ADVANCE_RANK: '0',
},
{
id: 'team8_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (4팀, 4팀)',
value: '8',
teams: [4, 4],
ADVANCE_RANK: '1',
},
{
id: 'team8_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (4팀, 4팀)',
value: '9',
teams: [4, 4],
ADVANCE_RANK: '2',
},
{
id: 'team6_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (3팀, 3팀)',
value: '10',
teams: [3, 3],
ADVANCE_RANK: '1',
},
{
id: 'team6_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (3팀, 3팀)',
value: '11',
teams: [3, 3],
ADVANCE_RANK: '2',
},
{
id: 'team6_3',
label: '한조 리그전 이후 1,2,3위팀 진출 토너먼트 (6팀)',
value: '12',
teams: [6],
ADVANCE_RANK: '3',
},
{
id: 'team14_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (3팀, 4팀, 3팀, 4팀)',
value: '13',
teams: [3, 4, 3, 4],
ADVANCE_RANK: '1',
},
{
id: 'team14_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (3팀, 4팀, 3팀, 4팀)',
value: '14',
teams: [3, 4, 3, 4],
ADVANCE_RANK: '2',
},
{
id: 'team14_3',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (4팀, 5팀, 5팀)',
value: '15',
teams: [4, 5, 5],
ADVANCE_RANK: '2',
},
{
id: 'team16_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (4팀, 4팀, 4팀, 4팀)',
value: '16',
teams: [4, 4, 4, 4],
ADVANCE_RANK: '1',
},
{
id: 'team16_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (4팀, 4팀, 4팀, 4팀)',
value: '17',
teams: [4, 4, 4, 4],
ADVANCE_RANK: '2',
},
{
id: 'team18_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (4팀, 5팀, 4팀, 5팀)',
value: '18',
teams: [4, 5, 4, 5],
ADVANCE_RANK: '1',
},
{
id: 'team18_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (4팀, 5팀, 4팀, 5팀)',
value: '19',
teams: [4, 5, 4, 5],
ADVANCE_RANK: '2',
},
{
id: 'team18_3',
label:
'조별 리그전 이후 1위팀 진출 토너먼트 (3팀, 3팀, 3팀, 3팀, 3팀, 3팀)',
value: '20',
teams: [3, 3, 3, 3, 3, 3],
ADVANCE_RANK: '1',
},
{
id: 'team20_1',
label: '조별 리그전 이후 1위팀 진출 토너먼트 (5팀, 5팀, 5팀, 5팀)',
value: '21',
teams: [5, 5, 5, 5],
ADVANCE_RANK: '1',
},
{
id: 'team20_2',
label: '조별 리그전 이후 1,2위팀 진출 토너먼트 (5팀, 5팀, 5팀, 5팀)',
value: '22',
teams: [5, 5, 5, 5],
ADVANCE_RANK: '2',
},
{
id: 'team20_3',
label:
'조별 리그전 이후 1위팀 진출 토너먼트 (3팀, 3팀, 4팀, 3팀, 3팀, 4팀)',
value: '23',
teams: [3, 3, 4, 3, 3, 4],
ADVANCE_RANK: '1',
},
];
const idCheck = (id: string) => {
const findOption = options.find(option => option.id === id);
const Len = findOption?.teams.length;
if (id === 'team6_3') return 3;
return Len;
};
// const Len = idCheck('여기 아이디')
const Len = 6;
const Level1 = () => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
<View style={styles.horizontalConnectorLevel1} />
<View style={styles.matchContainer}>
{/* 팀1 */}
<View style={styles.teamContainerLevel1}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
{Len === 2 ? (
<View style={styles.team}>
<Text>1조1위</Text>
</View>
) : (
<Level2 index={0} />
)}
</View>
{/* 팀2 */}
<View style={styles.teamContainerLevel1}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
{Len === 2 ? (
<View style={styles.team}>
<Text>2조1위</Text>
</View>
) : Len === 3 ? (
<OddLevel2 index={1} />
) : (
<Level2 index={1} />
)}
</View>
</View>
</>
);
};
const Level2 = ({index}) => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
<View style={styles.horizontalConnectorLevel2} />
<View style={styles.matchContainer}>
{/* 팀1 */}
<View style={styles.teamContainerLevel2}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
{Len < 5 && (
<View style={styles.team}>
{index === 0 ? <Text>1조1위</Text> : <Text>3조1위</Text>}
</View>
)}
{Len > 4 && <Level3 index={index} />}
</View>
{/* 팀2 */}
<View style={styles.teamContainerLevel2}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
{Len < 5 && (
<View style={styles.team}>
{index === 0 ? <Text>2조1위</Text> : <Text>4조1위</Text>}
</View>
)}
{Len > 4 && <OddLevel3 index={index} />}
</View>
</View>
</>
);
};
const OddLevel2 = ({index}) => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
{/* <View style={styles.horizontalConnectorLevel2} /> */}
<View style={styles.matchContainer}>
{/* 팀1 */}
{/* <View style={styles.teamContainerLevel2}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>팀3</Text> : <Text>팀5</Text>}
</View>
{Len > 4 && <Level3 />}
</View> */}
{/* 팀2 */}
<View style={styles.teamContainerLevel2}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>팀4</Text> : <Text>3조1위</Text>}
</View>
{Len > 4 && <OddLevel3 index={index} />}
</View>
</View>
</>
);
};
const Level3 = ({index}) => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.verticalConnector} />
{/* 중간 연결선 */}
<View style={styles.horizontalConnectorLevel3} />
<View style={styles.matchContainer}>
{/* 팀1 */}
<View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>1조1위</Text> : <Text>4조1위</Text>}
</View>
</View>
{/* 팀2 */}
<View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>2조1위</Text> : <Text>5조1위</Text>}
</View>
</View>
</View>
</>
);
};
const OddLevel3 = ({index}) => {
return (
<>
{/* 최종 승자로부터 뻗어내리는 세로 연결선 */}
<View style={styles.oddVerticalConnector} />
{/* 중간 연결선 */}
{/* <View style={styles.horizontalConnectorLevel3} /> */}
<View style={styles.matchContainer}>
{/* 팀1 */}
{/* <View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
<Text>팀1</Text>
</View>
</View> */}
{/* 팀2 */}
<View style={styles.teamContainerLevel3}>
<View style={[styles.verticalConnector, styles.halfConnector]} />
<View style={styles.team}>
{index === 0 ? <Text>3조1위</Text> : <Text>6조1위</Text>}
</View>
</View>
</View>
</>
);
};
const TestModal = ({
isVisible,
text,
secondText,
onConfirm,
onClose,
}: IProps) => {
return (
<Modal visible={isVisible} transparent={true} onRequestClose={onClose}>
{/* <FullScreenLoader isLoading={isLoading} /> */}
<Pressable onPress={onClose} style={{flex: 1}}>
<View style={styles.modalOverlay}>
{/* 모달의 콘텐츠 부분 */}
<View style={styles.modalView}>
<View style={styles.bracket}>
{/* 최종 승자 */}
<View style={styles.team}>
<Text>우승팀</Text>
</View>
<Level1 />
</View>
</View>
</View>
</Pressable>
</Modal>
);
};
const styles = StyleSheet.create({
modalOverlay: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'rgba(255, 255, 255, 1)', // 반투명 배경
},
modalView: {
position: 'absolute',
justifyContent: 'center',
alignItems: 'center',
// width: '60%', // 모달의 폭
// width: 'auto',
width: '100%',
height: 'auto', // 모달의 높이
backgroundColor: '#434242',
padding: 20,
borderRadius: 10,
shadowColor: '#000',
shadowOffset: {width: 0, height: 2},
shadowOpacity: 0.25,
shadowRadius: 4,
elevation: 5,
},
verticalGreyLine: {
width: 1,
height: '100%',
borderWidth: 0.5,
borderColor: colors.greyLine,
},
bracket: {
// backgroundColor: 'red',
width: 500,
flexDirection: 'column',
alignItems: 'center',
justifyContent: 'center',
marginTop: 50,
},
matchContainer: {
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'center',
},
teamContainerLevel1: {
width: 200,
// backgroundColor: 'darkblue',
flexDirection: 'column',
// justifyContent: 'space-between',
alignItems: 'center',
},
teamContainerLevel2: {
width: 100,
// backgroundColor: 'blue',
flexDirection: 'column',
// justifyContent: 'space-between',
alignItems: 'center',
},
teamContainerLevel3: {
width: 50,
// backgroundColor: 'blue',
flexDirection: 'column',
// justifyContent: 'space-between',
alignItems: 'center',
},
team: {
backgroundColor: 'green',
padding: 10,
borderWidth: 1,
borderColor: 'black',
marginVertical: 5,
},
horizontalConnectorLevel1: {
borderTopWidth: 1,
borderColor: 'black',
width: width,
},
horizontalConnectorLevel2: {
borderTopWidth: 1,
borderColor: 'black',
width: width / 2,
},
horizontalConnectorLevel3: {
borderTopWidth: 1,
borderColor: 'black',
width: width / 4,
},
verticalConnector: {
borderLeftWidth: 1,
borderColor: 'black',
minHeight: width / 6,
},
oddVerticalConnector: {
borderLeftWidth: 1,
borderColor: 'black',
minHeight: width / 6,
},
halfConnector: {
minHeight: 25,
},
});
export default TestModal;
'RN' 카테고리의 다른 글
자식 컴포넌트에서 함수나 상태 끌어올리기 (0) | 2023.12.10 |
---|---|
기본적인 모달 (0) | 2023.12.10 |
stack 넘어갈때 화면 번쩍임 해결(흰화면) (0) | 2023.12.08 |
FlatList 격자형태로 이쁘게 나열하기 (0) | 2023.12.08 |
style 따로 빼서 쓰기 (0) | 2023.12.07 |